Crate binaryninja
source ·Expand description
This crate is the official Binary Ninja API wrapper for Rust.
Binary Ninja is an interactive disassembler, decompiler, and binary analysis platform for reverse engineers, malware analysts, vulnerability researchers, and software developers that runs on Windows, macOS, and Linux. Our extensive API can be used to create and customize loaders, add or augment architectures, customize the UI, or automate any workflow (types, patches, decompilation…anything!).
If you’re just getting started with Binary Ninja, you may wish to check out the Getting Started Guide
If you have questions, we’d love to answer them in our public Slack, and if you find any issues, please file an issue or submit a PR.
§Warning
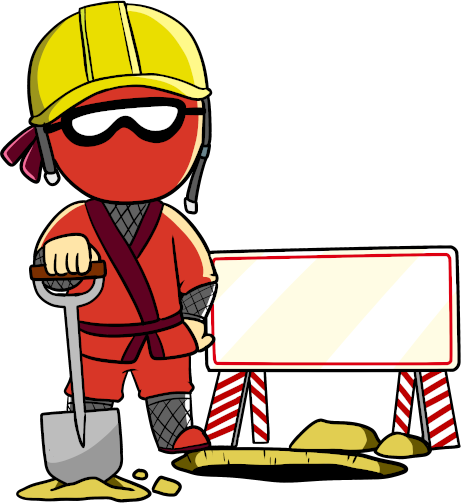
⚠️ These bindings are in a very early beta, only have partial support for the core APIs and are still actively under development. Compatibility will break and conventions will change! They are being used for core Binary Ninja features however, so we expect much of what is already there to be reliable enough to build on, just don’t be surprised if your plugins/scripts need to hit a moving target.
⚠️ This project runs on Rust version
1.77.0
§Examples
There are two distinct ways to use this crate:
§Writing a Plugin
Create a new library (cargo new --lib <plugin-name>
) and include the following in your Cargo.toml
:
[lib]
crate-type = ["cdylib"]
[dependencies]
binaryninja = {git = "https://github.com/Vector35/binaryninja-api.git", branch = "dev"}
In lib.rs
you’ll need to provide a CorePluginInit
or UIPluginInit
function for Binary Ninja to call.
See command
for the different actions you can provide and how to register your plugin with Binary Ninja.
§Writing a Script:
“Scripts” are binaries (cargo new --bin <script-name>
), and have some specific requirements:
§build.rs
Because the only official method of providing linker arguments to a crate is through that crate’s build.rs
, all scripts need to provide their own build.rs
so they can probably link with Binary Ninja.
The most up-to-date version of the suggested build.rs
is here.
§main.rs
Standalone binaries need to initialize Binary Ninja before they can work. You can do this through headless::Session
, headless::script_helper
, or headless::init()
at start and headless::shutdown()
at shutdown.
// This loads all the core architecture, platform, etc plugins
// Standalone executables need to call this, but plugins do not
let headless_session = binaryninja::headless::Session::new();
println!("Loading binary...");
let bv = headless_session.load("/bin/cat").expect("Couldn't open `/bin/cat`");
// Your code here...
§Cargo.toml
[dependencies]
binaryninja = { git = "https://github.com/Vector35/binaryninja-api.git", branch = "dev"}
See the examples on GitHub for more comprehensive examples.
Modules§
- Architectures provide disassembly, lifting, and associated metadata about a CPU to inform analysis and decompilation.
- Background tasks provide plugins the ability to run tasks in the background so they don’t hand the UI
- A convenience class for reading binary data
- A view on binary data and queryable interface of a binary file.
- A convenience class for writing binary data
- Contains and provides information about different systems’ calling conventions to analysis.
- Provides commands for registering plugins and plugin actions.
- An interface for providing your own BinaryViews to Binary Ninja.
- A basic wrapper around an array of binary data
- Parsers and providers of debug information to Binary Ninja.
- Interfaces for demangling and simplifying mangled names in binaries.
- Interfaces for creating and displaying pretty CFGs in Binary Ninja.
- Interfaces for asking the user for information: forms, opening files, etc.
- APIs for accessing Binary Ninja’s linear view
- To use logging in your script, do something like:
- Contains all information related to the execution environment of the binary, mainly the calling conventions used
- Reference counting for core Binary Ninja objects.
- Sections are crate::segment::Segments that are loaded into memory at run time
- Labeled segments in a binary file that aren’t loaded in to memory
- An interface for reading, writing, and creating new settings
- String wrappers for core-owned strings and strings being passed to the core
- Interfaces for the various kinds of symbols in a binary.
- Interfaces for creating and modifying tags in a BinaryView.
Structs§
Enums§
Traits§
- The trait required for receiving core object destruction callbacks.
Functions§
- The main way to open and load files into Binary Ninja. Make sure you’ve properly initialized the core before calling this function. See
crate::headless::init()
- The main way to open and load files (with options) into Binary Ninja. Make sure you’ve properly initialized the core before calling this function. See
crate::headless::init()